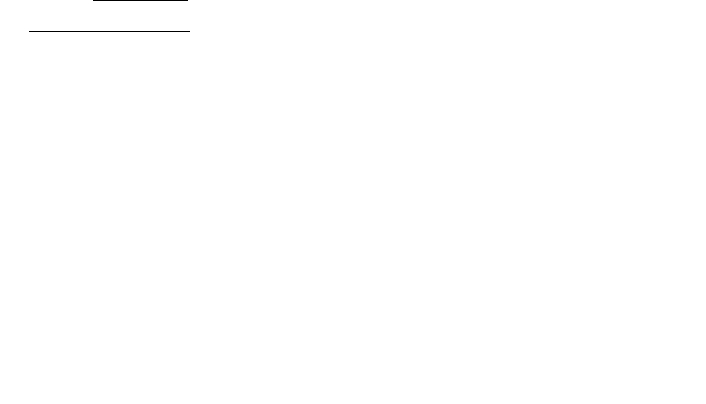
Decisions in Java – The IF Statement
Exercises
1. Write Java statements to perform each task.
(a) Add one to the value of zeroCount if the variable total has the value zero.
(b) Add one to the value of pageCount if the variable lineCount is greater than pageLength.
(c) Set the value of the boolean variable leftSide to true if the int variable page is even, and to
false if page is odd.
(d) Determine if the the int variable n is a perfect square and then display an appropriate
message. (difficult question)
2. Write a program that will ask the user for two int values and determine which one has the greatest
value (i.e., higher on the number line). The program should output something like, "The number x
has the greatest value", where x is one of the numbers.
3. Write a program that will ask the user for two int values and determine if the first divides evenly
into the second, displaying a message depending on the result. For example, the number 2
divides evenly into 4, but 4 does not divide into 2 (so order matters). Hint: You may find the
modulo operator (%) useful here.
4. Write a program that will ask the user for two float values and compare their magnitudes. For
example, if the user entered 3 and -9, the program should respond that -9 has the greater
magnitude.
5. Write a program that solves an equation of the form
. The program should prompt the
user for values of a and b, then solve the equation for x and print the results. The program should
take appropriate action if a is zero.
6. A quadratic equation in the form
has roots (or zeroes) given by
Write a program that determines the values of the roots, root1 and root2, of the quadratic
equation after asking the user to input values for a, b, and c. The program should take show
appropriate messages if:
(a) the value of a is zero (in which case, see Exercise #4), and
(b) there are two, one, or zero real roots (you cannot take the square root of a negative
number).
Hint: There are two roots for the quadratic, which can be determined using the following code.
Page 4 of 7
root1 = (-1 * b + Math.sqrt(b^2 – 4 * a * c)) / (2 * a);
root1 = (-1 * b - Math.sqrt(b^2 – 4 * a * c)) / (2 * a);